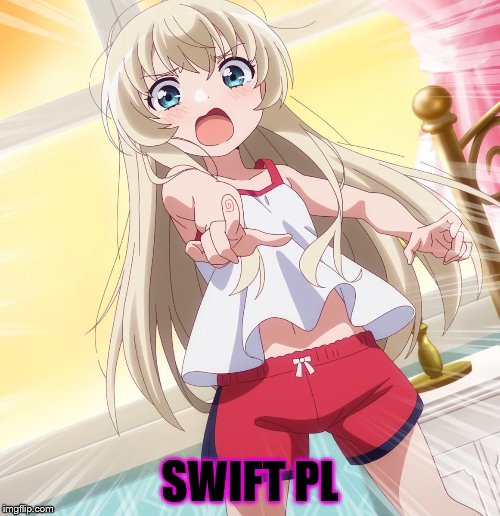
online swift playground :
http://online.swiftplayground.run/
on a macOS 10.12.6 or later device DL latest versions of both the iOS SDK and Xcode can be
downloaded free of charge from the Mac App Store (search and install xcode)
add your appleID to your Xcode :
Xcode -> Preferences... menu option and select the Accounts tab
On the Accounts screen, click on the + button select Apple IDfrom the resulting panel, continue
When prompted, enter your Apple ID and associated password and click on the Sign In
button to add the account to the preferences
enroll as a developer, and get access to forums and test devices :
https://developer.apple.com/programs/enroll/
Developer(not enrolled as a developer) and Distribution(if you enrolled as a developer)
Signing Identities :
view current signing identities : select the
newly added Apple ID in the Accounts panel and click on the Manage Certificates
To create the iOS Development signing identity : click on the + button and choose
iOS dev or distribution option
OOP :
declare a class : class NewClassName{}
open Xcode, Create a new Xcode project, single view app :
development team : if you havent enrolled as an Apple developer program set to none
Organization Name field : used for copyrights
company identifier = reverse URL : com.yotamrker
set PL(prog lang) to swift
the Main.storyboard file is the UI screen to drag drop controllers into
swift online IDE : http://online.swiftplayground.run/
or from Xcode choose open playgraound which is an IDE for testing code snippets
and practicing swift
make Xcode dark theme : toolbar, Xcode, pref, fonts and colors
declare var :
the compiler deduces var type
var mynumber = 10
var myChar4 = "\u{0058}"
explicit var declare : var x : Int = 10
print("Int32 Min= \(Int32.min) Int32 Max = \(Int32.max)")
outputs : Int32
Min = -2147483648 Int32 Max = 2147483647
const : let maxCount = 100
string megazord :
var message = "\(userName) has \(inboxCount) message. Message capacity remaining is \(maxCount -inboxCount)"
in the () are variables
output :
print(message)
declare const : let bookTitle: String
conditional : if Bool {} else{}
simple array program :
simple grade alg with avg, sum :
swift code (run at swift online IDE : http://online.swiftplayground.run/)
Code:
Code:
import Foundation
var grades = [10, 20, 30, 40,50,60,70,80,100,100]
var students = ["one","two","three","four","five","roku","shichi","hachi","kyuu","juu"]
let fail = 55
var sum=0
var max = 0
print(grades.count)
for idx in 0...grades.count-1
{
sum+=grades[idx]
if max <= grades[idx] {max = grades[idx]}
if grades[idx] < fail {print("\(students[idx]) has failed")}
}
var avg = sum/grades.count
print("avg grade : \(sum/grades.count)")
print("highest ranking students :")
for idx in 0...grades.count-1
{
if max == grades[idx] {print(students[idx])}
}
print("below avg :")
for idx in 0...grades.count-1
{
if avg > grades[idx] {print(students[idx])}
}
out put :
10
one has failed
two has failed
three has failed
four has failed
five has failed
avg grade : 56
highest ranking students :
kyuu
juu
below avg :
one
two
three
four
five
optional var (could be nil (null)):
var index: Int?
index = 3
var treeArray = ["Oak", "Pine", "Yew", "Birch"]
if index!=nil{print(treeArray[index!])}else{print("nil index")}
to declare a var when you know it will have a value no matter what :
var index: Int! // Optional is now implicitly unwrapped
index = 3
var treeArray = ["Oak", "Pine", "Yew", "Birch"]
if index != nil {print(treeArray[index])} else {print("index doesnot contain a value")}
var multiline = """
digimon
digital monsters, digimon
are the champions !
"""
print(multiline)
special chars :
var newline = "\n"
var backslash = "\\"
for loop :
for i in 1...20{}
comments : // single line
/*
multiline comment
*/
let tuple = (1,"hi",2.5) // tuple var
print(tuple.2)
or
let tuple = (num:1,str:"hi",perc:2.5) // tuple var
print(tuple.num)
or
let tuple = (var1,var2,2.5) // tuple var
var x:Int? // optional var may be nil (null) or something
if x != nil{}
else{}
var y:String?
y = "shouryuken"
var x = ""
x = y! // y converted to string type (unwrapped)
print(x)
or
var y:String?
y = "shouryuken"
var x = ""
if let x = y {print(x)} // as unwrapped
if let y = y, true == true {print(x)} // multi conditional if
as! String // type upcasting
let myButton: UIButton = UIButton()
let myControl = myButton as UIControl // downcasting
check var type :
if myobject is MyClass {
// myobject is an instance of MyClass
}
operators * / + - % (remainder)
short operators :
x %= y
Comparison Operators :
as in java : == > < >= !=
Boolean Logical Operators :
or (||) and (&&) xor (^) not (!)
Range Operators :
x..y
x..<y
one range :
x...
...y
ternary expression(shortened if) :
print("Largest number is \(x > y ? x : y)")
bitwise operator
bit inverter :
let z = ~y
convert 0101 to 1010
no ref loop :
var c = 0
for _ in 1...5 {c +=1}
while loop :
while bool {}
repeat{//statement}
while bool
bresk loop : break
continue loop : continue // jumps to start of next loop
guard <boolean expressions> else {// code to be executed if expression is false<exit statement here>}
// code here is executed if expression is true
import Foundation
func multiplyByTen(value: Int?) {
guard let number = value, number < 10 else {
print("Number is too high")
return
}
let result = number * 10
print(result)
}
multiplyByTen(value:11)
the guard can only live in a function because it has to have a return in its else
switch :
func simpleSwitch(value: Int) {
switch value
{
case 1:
print("one")
case 2:
print("")
case 3,4:
print("3 or 4")
default:
print("case else")
}
}
simpleSwitch(value:1)
a case can also contain a range : case 3...9:
limit the case further : case 3...9 where value == 6:
if no action needed for the default case :
default:
break
methodes :
void function :
func sayHello() {print("Hello")}
sayHello() // summon func
func with params and return :
func buildMessageFor(name: String, count: Int) -> String {
return("\(name), you are customer number \(count)")
}
let message = buildMessageFor(name: "moti", count: 100)
print(message)
use _ when you dont care what the function returns
func buildMessageFor(name: String, count: Int) -> String {
print("moti rulz")
return("\(name), you are customer number \(count)")
}
_ = buildMessageFor(name: "moti", count: 100)
field names exclution in function params :
func buildMessageFor(_ name: String,_ count: Int) -> String {
print("moti rulz")
return("\(name), you are customer number \(count)")
}
_ = buildMessageFor("moti", 100)
you can also replace _ with your preffered name, then summon :
buildMessageFor(x:"John", y:100)
a param can be declared with a default in case you want to pass only one param :
func buildMessageFor(_ name: String = "client", count: Int) -> String {
print("moti rulz")
return("\(name), you are customer number \(count)")
}
print(buildMessageFor(count:100))
returning several vars using a tuple :
Code:
func sizeConverter(_ length: Float) -> (yards: Float, centimeters: Float,meters: Float) {
let yards = length * 0.0277778
let centimeters = length * 2.54
let meters = length * 0.0254
return (yards, centimeters, meters)
}
let lengthTuple = sizeConverter(20)
print(lengthTuple.yards)
print (lengthTuple.centimeters)
print(lengthTuple.meters)
variadic parameters function param :
func displayStrings(_ strings: String...)
{
for string in strings {
print(string)
}
}
displayStrings("one", "two", "three", "four")
sending param by ref :
func doubleValue (_ value: inout Int) -> Int {
value += value
return(value)
}
var myValue = 2
print("doubleValue call returned \(doubleValue(&myValue))")
print(myValue)
POSO class example :
Code:
import Foundation
class BankAccount{
var accountBalance: Float = 0
var accountNumber: Int = 0
// c'tor :
init(number: Int, balance: Float)
{
accountNumber = number
accountBalance = balance
}
deinit{
// code to run when the object is destroyed
}
func displayBalance(){
print("Number \(accountNumber)")
print("Current balance is \(accountBalance)")}
class func getMaxBalance() -> Float {
return 100000.00} // static function
}
// var account1: BankAccount = BankAccount() // usable without constructor (default c'tor)
var account1: BankAccount = BankAccount(number:1,balance: 1005)
account1.accountBalance = 2005 // access var in the class
account1.displayBalance()
print(BankAccount.getMaxBalance())
getters and setters :
class BankAccount {
var accountBalance: Float = 0
var accountNumber: Int = 0;
let fees: Float = 25.00
var balanceLessFees: Float {get {return accountBalance - fees}
set(newBalance){accountBalance = newBalance - fees}}
init(number: Int, balance: Float)
{
accountNumber = number
accountBalance = balance
}
}
var account1: BankAccount = BankAccount(number:1,balance: 1005)
var balance1 = account1.balanceLessFees // input from getter
print(balance1)
account1.balanceLessFees = 3000
print(account1.balanceLessFees)// output from setter
self (in java this. ) :
class MyClass {
var myNumber = 1
func addTen() {
self.myNumber += 10
}
}
var x = MyClass()
x.addTen()
print(x.myNumber) // output 11
// inheritance example
class BankAccount{
var accountBalance: Float = 0
var accountNumber: Int = 0
// c'tor :
init(number: Int, balance: Float)
{
accountNumber = number
accountBalance = balance
}
func displayBalance(){
print("Number \(accountNumber)")
print("Current balance is \(accountBalance)")}
}
class SavingsAccount: BankAccount {
var interestRate: Float = 0.0
func calculateInterest() -> Float{return interestRate * accountBalance}
}
var x = SavingsAccount(number: 10, balance:20)
x.interestRate = 5
print(x.calculateInterest())
*********************************************************************************
Code:
import Foundation
// inheritance with override
class BankAccount{
var accountBalance: Float = 0
var accountNumber: Int = 0
// c'tor :
init(number: Int, balance: Float)
{
accountNumber = number
accountBalance = balance
}
func displayBalance(){
print("Number \(accountNumber)")
print("Current balance is \(accountBalance)")}
}
class SavingsAccount: BankAccount {
var interestRate: Float = 0.0
func calculateInterest() -> Float{return interestRate * accountBalance}
override func displayBalance(){
print("Number \(accountNumber)")
print("Current balance is \(accountBalance)")
print("Prevailing interest rate is \(interestRate)")}
}
var x = SavingsAccount(number: 10, balance:20)
x.interestRate = 5
x.displayBalance()
**********************************************************************************
override methode with super :
override func displayBalance(){
super.displayBalance()
print("Prevailing interest rate is \(interestRate)")
}
}
************************************************************************
subclass c'tor + initializing its instance :
import Foundation
// inheritance with override
class BankAccount{
var accountBalance: Float = 0
var accountNumber: Int = 0
// c'tor :
init(number: Int, balance: Float)
{
accountNumber = number
accountBalance = balance
}
func displayBalance(){
print("Number \(accountNumber)")
print("Current balance is \(accountBalance)")}
}
class SavingsAccount: BankAccount {
var interestRate: Float = 0.0
init(number: Int, balance: Float, rate: Float)
{
interestRate = rate
super.init(number: number, balance: balance)
}
func calculateInterest() -> Float{return interestRate * accountBalance}
override func displayBalance(){
super.displayBalance()
print("Prevailing interest rate is \(interestRate)")
}
}
var x = SavingsAccount(number: 12311, balance: 600.00, rate: 0.07)
x.interestRate = 5
x.displayBalance()
**********************************************************************************
add jutsu to any class :
extension ClassName {
// new features here
}
usage :
import Foundation
// inheritance with override
extension Double {
var squared: Double {
return self * self}
var cubed: Double {
return self * self * self}
}
// usage :
let myValue: Double = 3.0
print(myValue.squared)
***********************************************************
array :
var treeArray = ["Pine", "Oak", "Yew"]
var treeArray: [String] = ["Pine", "Oak", "Yew"]
var variableName = [type]() // empty array
var nameArray = [String](repeating: "My String", count: 10) // a 10 sized array
// with each organ containing "My String"
let firstArray = ["Red", "Green", "Blue"]
let secondArray = ["Indigo", "Violet"]
// monster fusion spell card :
let thirdArray = firstArray + secondArray
get array count : arrayVar.count
is array empty Bool : .isEmpty
get specific organ in the array : arrayVar[2]
set organ : arrayVar[1] = "Redwood"
********************************************
append to array :
var treeArray = ["Pine", "Oak", "Yew"]
treeArray.append("Redwood")
treeArray += ["Redwood"]
treeArray += ["Redwood", "Maple", "Birch"]
print(treeArray) // output : ["Pine", "Oak", "Yew", "Redwood", "Redwood", "Redwood", "Maple", "Birch"]
array tricks : treeArray.insert("Maple", at: 0)
treeArray.remove(at: 2)
treeArray.removeLast()
array loop (object loop):
let treeArray = ["Pine", "Oak", "Yew", "Maple", "Birch", "Myrtle"]
for tree in treeArray {print(tree)}
**********************************************************
mixed object array :
let mixedArray: [Any] = [1, 2, 45, "Hello"]
**********************************************************
var casting and Any type var :
let x :Any?
x = 10
var y = x as! Int
print(y + 1)
*****************************************************************************
Dictionary type var :
var bookDict = ["battle programming grimoire": "30$",
"xamarin grimoire" : "20$", "android gromoire" : "25$"]
print(bookDict)
OR :
// declare using type annotation :
var bookDict: [String: String] = ["battle programming grimoire": "30$",
"xamarin grimoire" : "20$", "android gromoire" : "25$"]
print(bookDict)
OR :
var myDictionary = [Int: String]() // declare an empty dictionary
*************************************************************************8
Sequence - based Dictionary Initialization
let keys = ["battle programming grimoire","book2","book3"]
let values = [30,20,25]
let bookDict = Dictionary(uniqueKeysWithValues: zip(keys, values))
print(bookDict)//output :
// ["book2": 20, "book3": 25, "battle programming grimoire": 30]
the above zip can use ranges :
let keys = ["battle programming grimoire","book2","book3"]
let bookDict = Dictionary(uniqueKeysWithValues: zip(keys, 1...))
print(bookDict)//output :
// ["book3": 3, "battle programming grimoire": 1, "book2": 2]
bookDict.count // gets size of dictionary
bookDict[key] // get organ
print(bookDict["hadouken", default: 9001]) // get organ of default val if not found
set dictionary organ val :
bookDict["200-532874"] = "Sense and Sensibility"
OR :
bookDict.updateValue("The Ruins", forKey: "200-532874")
add or remove a dictionary organ :
import Foundation
let keys = ["battle programming grimoire","book2","book3"]
var bookDict = Dictionary(uniqueKeysWithValues: zip(keys, 1...))
bookDict["300-898871"] = 4 // add a key
print(bookDict)
bookDict.removeValue(forKey: "300-898871")
print(bookDict)
dictionary iteration :
for (bookid, title) in bookDict {
print("Book ID: \(bookid) Title: \(title)")}
***************************************************************************
err handling :
Code:
// some global vars :
let connectionOK = true
let connectionSpeed = 31.00
let fileFound = true
// an enum of errs
enum FileTransferError: Error {
case noConnection
case lowBandwidth
case fileNotFound
}
// a fuction that may throw an err
func fileTransfer() throws -> Bool{
guard connectionOK else {throw FileTransferError.noConnection}
guard connectionSpeed > 30 else {throw FileTransferError.lowBandwidth}
guard fileFound else {throw FileTransferError.fileNotFound}
return true
}
// using a throuse func
func sendFile() -> String {
do {try fileTransfer()} catch FileTransferError.noConnection {return("No Network Connection")}
catch FileTransferError.lowBandwidth {return("File Transfer Speed too Low")}
catch FileTransferError.fileNotFound {return("File not Found")}
catch {return("Unknown error")}
return("Successful transfer")}
print(sendFile())
Code:
if you know an err wont arise :
func sendFile() -> String {
try! fileTransfer() // you know it won't cause an error but the
//func you're using has throws in the signature
defer :
func sendFile() -> String {
defer {print("code to run right before return")}
try! fileTransfer() // you know it won't cause an error but the func you're using has throws in the signature
return("Successful transfer")}
print(sendFile())
// output :
// code to run right before return
// Successful transfer
hadouken :tokushushoukan